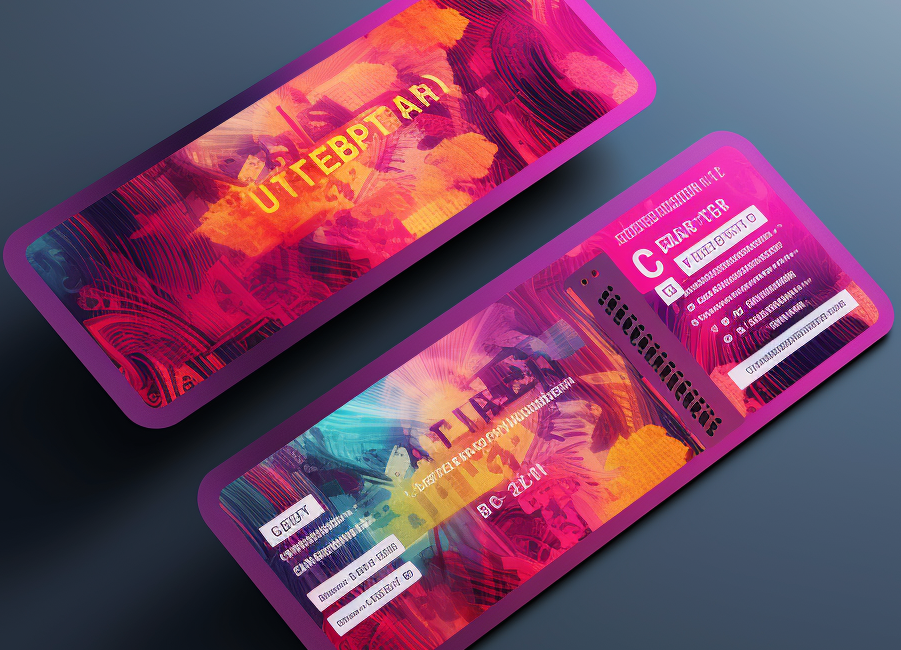
Decentralized NFT Marketplace | Part 1
In this article, we will look at what it is:
- What is decentralization?
- What does the NET Marketplace consist of?
- What role does NFT play?
- Creating a collection
- We sell TAE on opensea
- Sources?
Decentralization
In simple words, it is like an online marketplace for digital unique things, where there is no single owner or management company. All transactions are carried out on the basis of "smart contracts" on the blockchain, which makes the process more transparent and ensures the security of transactions. Participants can buy, sell and exchange their unique digital assets without intermediate intermediaries, which emphasizes the idea of decentralization.
What is the difference between decentralization and centralization?
Centralization: Everything is concentrated in one place, where there is a “boss” or control center who makes the main decisions. The rest simply follow the instructions of this "boss".
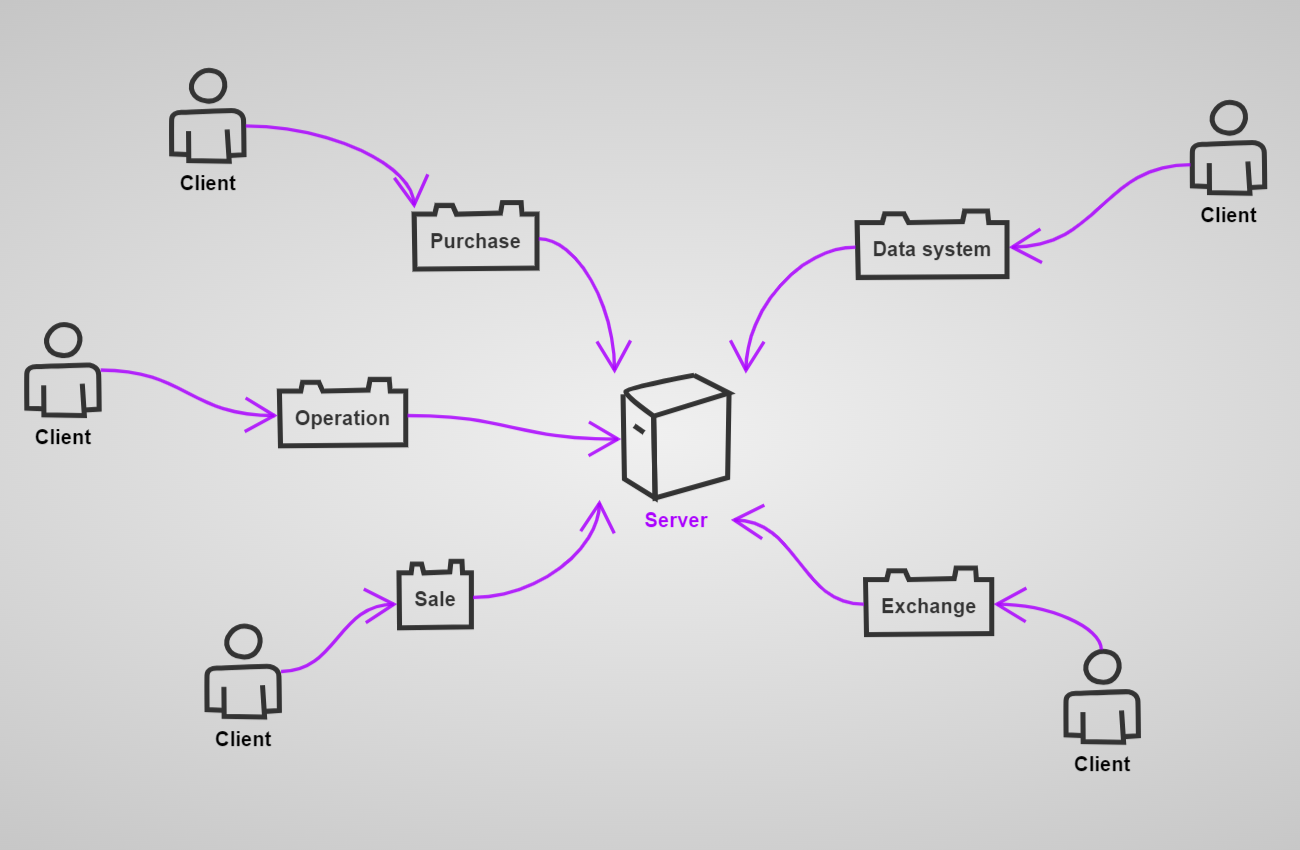
Decentralization: Everything is divided into parts, each of which works independently. There is no one big “boss” who decides everything. Everyone does their part
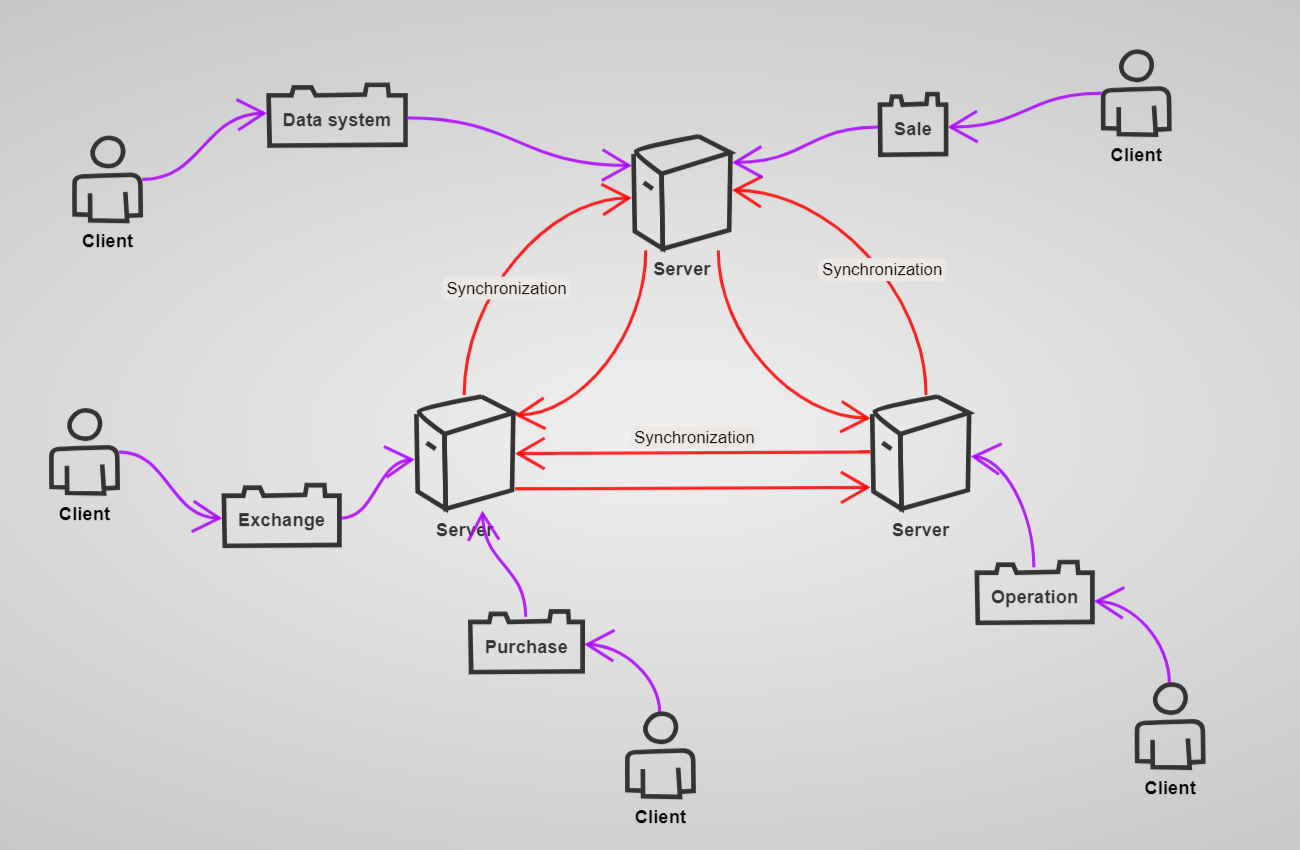
NFT Marketplace
The NFT Marketplace consists of several key contracts that enable various aspects of the functioning of the digital unique asset market. Here are the main components of the NFT Marketplace:
- NFT contract (eg ERC-721): Responsible for creating and managing unique tokens (NFTs), defining their properties and tracking ownership.
- Marketplace Contract: Provides marketplace functionality including listing, buying, and selling NFTs. Associated with the NFT contract and manages transactions
- Payment management contract: Processes payments for NFT sales, accepts funds from buyers and pays sellers
All of these contracts interact with each other to provide the functionality of the NFT Marketplace, from creating unique tokens to conducting transactions and managing various aspects of the platform.
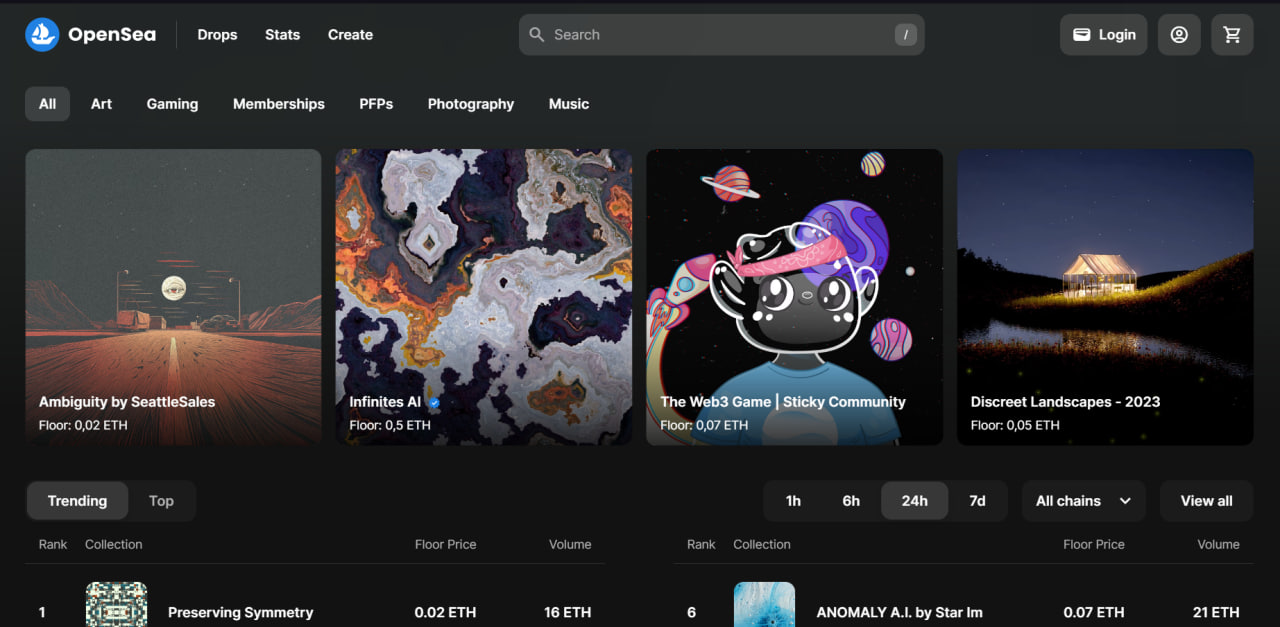
NFT in the world of decentralization
NFT- it's like a digital ticket confirming that you have something unique on the Internet. It could be digital picture, video, music or something else. When you buy NFT, you are essentially buying a record in a database that says you are the owner of this unique digital content. It's like having an artist's autograph, only in digital form
At certain sites NFT may provide additional bonuses, access to a unique resource, or increased income.
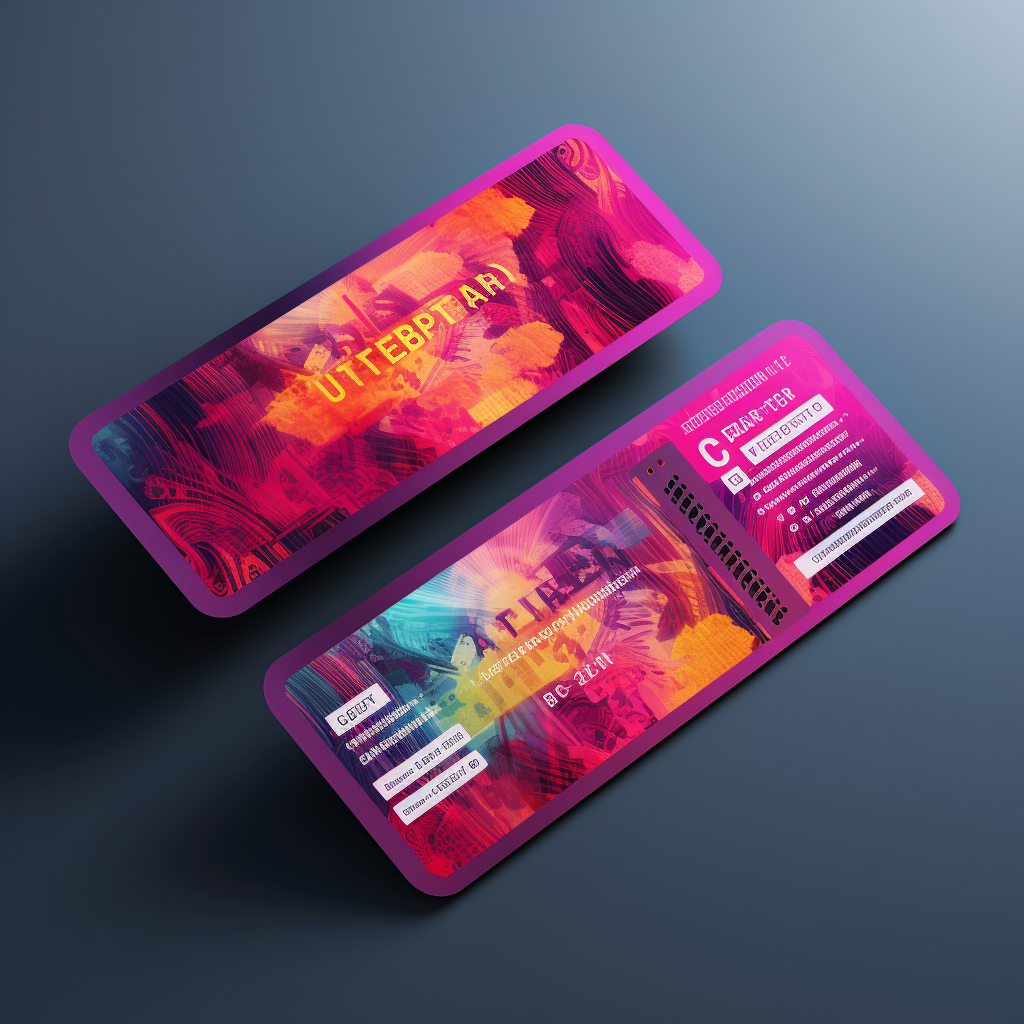
Collection
Collection - it's like your digital gallery or a set of unique digital things on the Internet. Instead of, to hold physical items, you collect unique digital files, such as pictures, videos or music, that are captured as NFTs. Each item in your collection has its own uniqueness and authenticity, confirmed by a record in the blockchain. It's like a virtual gallery of your digital treasures.
To create a collection, you can use ready-made solutions, which are huge. In this article I would like to dive deeper into this process, and I will begin our dive with two components:
Smart solidity contract
Solidity contract- who will be responsible for creating a collection and adding your own to this collection NFT.
All links to source code will be provided at the end of the article.
Let's write our own collection contract that will store our assets:
We create a contract and inherit from EIP ERC721
contract Collection {
constructor(
string memory name_,
string memory symbol_
) ERC721(name_, symbol_) {}
}
- constructor(string memory name_, string memory symbol_): This is a constructor function that is executed once when a smart contract instance is created. It accepts two strings (name_ and symbol_) that represent the name and symbol for our NFTs
- ERC721(name_, symbol_): This indicates that our Collection smart contract inherits ERC-721 functionality. ERC-721 is a standard that defines the basic functionality for creating unique and non-fungible tokens (NFTs) on the Ethereum blockchain.
- Thus, when creating a new instance of the Collection smart contract, we specify its name and symbol, and this contract will work with non-fungible tokens in accordance with the ERC-721 standard.
We create a token counter - this will be our unique ID for the token
import "@openzeppelin/contracts/utils/Counters.sol";contract Collection {
///LIBRARIES
using Counters for Counters.Counter;///INIT VAR
Counters.Counter private _tokenId;///...
- import "@openzeppelin/contracts/utils/Counters.sol";: This line of code imports the Counters library from
OpenZeppelin. The Counters library contains tools for working with counters and will be used to create unique token IDs - using Counters for Counters.Counter;: This line tells the smart contract that it will use functionality from the Counters library to operate on an object of type Counters.Counter. This is done in order to use the methods and variables defined in the library with the object _tokenId.
- Counters.Counter private _tokenId;: This is the definition of the _tokenId variable of type Counters.Counter. This counter will be used to assign unique IDs to each generated token in the collection. The private keyword indicates that this variable is only accessible within this
smart contract.
This allows the contract to create new tokens with unique numbers and track their supply.
Adding a token minting function to the contract
function mint(address to, string calldata tokenURI) external {
_mint(to, _tokenId._value);
_setTokenURI(_tokenId._value, tokenURI);
_tokenId.increment();
}
- function mint(address to, string calldata tokenURI) external {: This is a declaration of a function called mint that takes two parameters: to (the address where the new token will be sent) and tokenURI (the unique resource identifier for the new token). The external keyword indicates that the function can be called from outside the smart contract.
- _mint(to, _tokenId._value);: This line of code calls the _mint function, which is provided by the ERC-721 standard and adds a new token to the collection. The first argument to indicates who owns the new token, and the second argument _tokenId._value indicates the unique identifier of the new token.
- setTokenURI(_tokenId._value, tokenURI);: This line of code calls the _setTokenURI function, which sets the unique resource identifier for the new token. The first argument _tokenId._value specifies for which token the URI is being set, and the second argument tokenURI is the actual URI of the new token.
- _tokenId.increment();: This line of code increments the _tokenId counter, ensuring that the next token created will receive a new unique ID.
The mint function creates new unique tokens, assigns them unique identifiers, and sets their corresponding URIs in your ERC-721 collection.
Client for interaction with solidity contract
As a client, I chose the ethers web library. Instead of the client, you can use any other solution
Signer initialization
const signer: JsonRpcSigner = await new ethers.providers.Web3Provider(
window.ethereum
).getSigner();
Signer provides the ability to sign Ethereum transactions using your private key. Private key- This is an important part of your wallet and is used to prove that you own a specific Ethereum address.
Thus, Signer in ethers allows you to sign transactions, which is a necessary step to send funds or perform other operations on the Ethereum blockchain
Initializing the contracts instance
const address = "0x16f865b5Ce0B9894167bAFF999f86c7D47AB242f"
// The ABI can be obtained by compiling the contract or by reference
// https://gist.githubusercontent.com/kobylinskii-m/caef8024baab42cec220f0c51db640ac/raw/f14b72b78889d013a7f0c237e20e3398b91c73bf/Collection%2520abi
const abi = []
const contract = new ethers.Contract(address, abi, signer);
new ethers.Contract - creates a virtual connection between your code and a smart contract that exists on the blockchain
When you use new ethers.Contract, you specify the address of the smart contract and its ABI (interaction interface). An ABI is a set of rules with which your code can interact with a smart contract.
The created object then allows you to call smart contract functions from your code and receive results and events from the smart contract.
Calling the token chicank method
// The address of the token recipient
const to = "0x0Dc6bc1545A35644B8e082C76338ccF184703e55"
// Metadata Standards.
// More information: https://docs.opensea.io/docs/metadata-standards
const tokenURI = {
"description": "Friendly OpenSea Creature that enjoys long swims in the ocean.",
"external_url": "https://openseacreatures.io/3",
"image": "https://storage.googleapis.com/opensea-prod.appspot.com/puffs/3.png",
"name": "Dave Starbelly"
}
return await contract.mint(to, tokenURI);
When you use the functionmint, you generate a new unique token and give it properties that make it unique within this standard. This process is similar to how a bank issues new banknotes with unique numbers.
So the functionmint ERC-721 allows you to create and add new unique digital assets to a collection of non-fungible tokens (NFTs)
Opensea
OpenSea is an online marketplace for digital collectibles such as unique digital artificial works, video game items and other unique digital assets. Here, people can buy, sell and exchange these unique digital items using Ethereum blockchain technology and standards such as ERC-721 for non-fungible tokens (NFTs)
To create a collection, I will use already - demo clien
Creating a collection
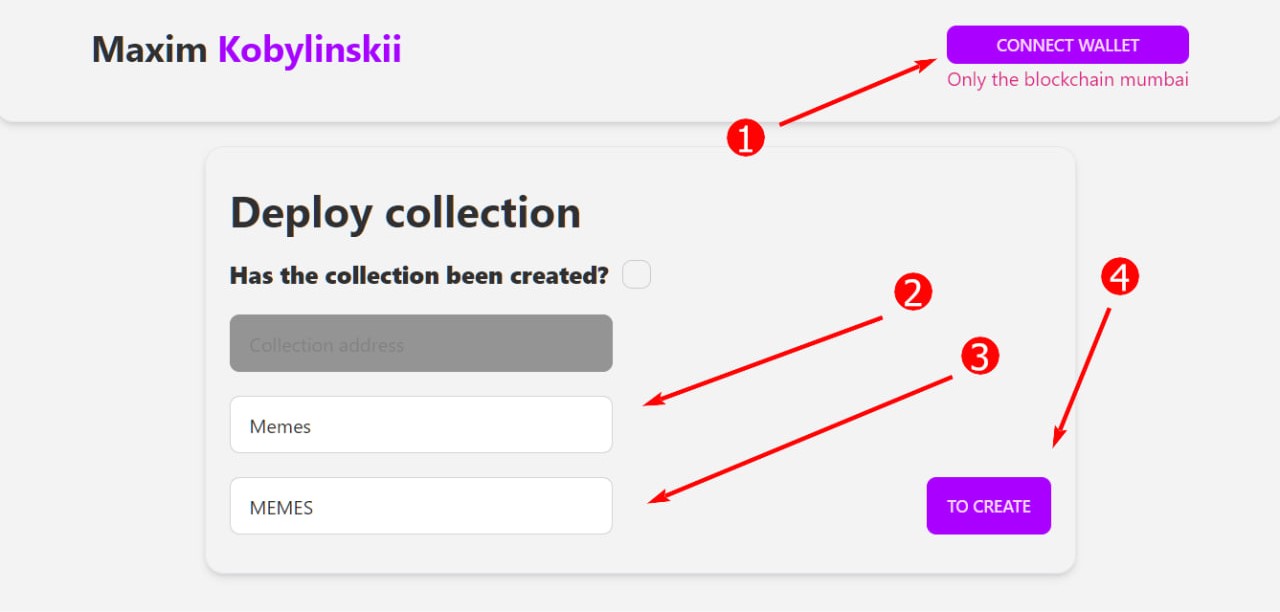
- Connect your metamask wallet and select the network MUMBAI
- Enter a name for the collection. The name will be displayed on the main page of the collection
- Enter symbol for the collection.
- Click create collection and confirm
Mintim tokens to the collection
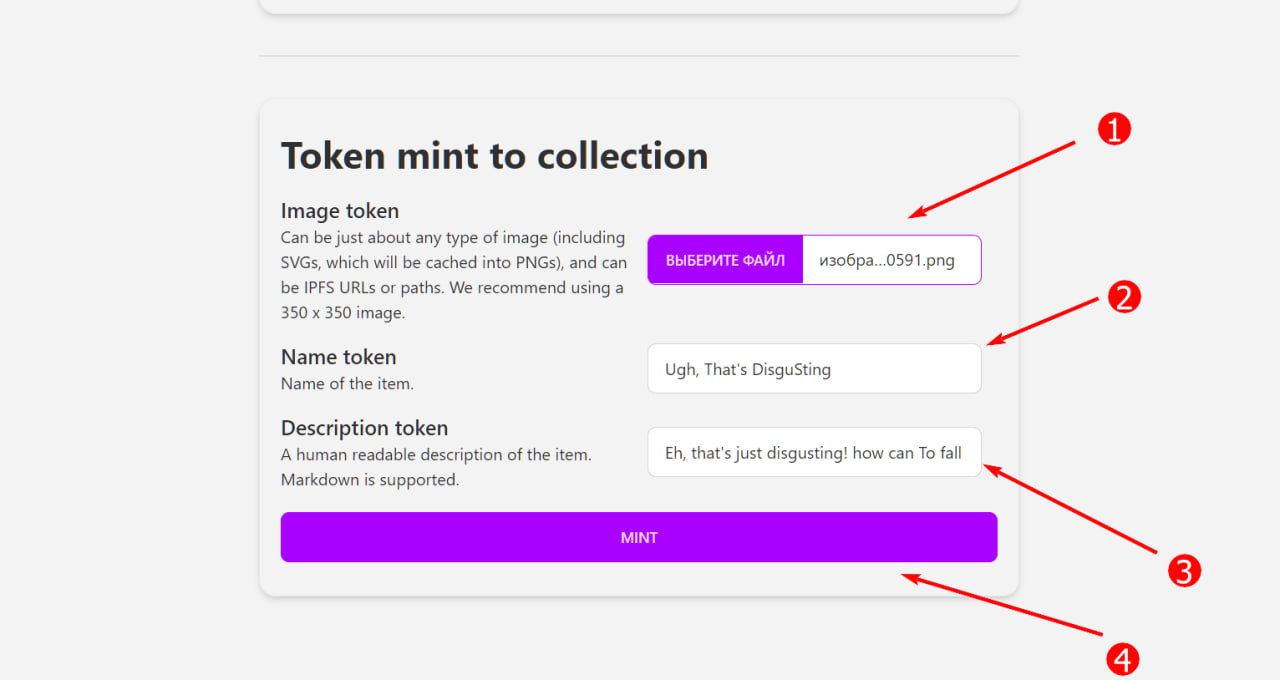
- Select the token image
- Name for the token
- Description of the token
- Click mint token and confirm the transaction
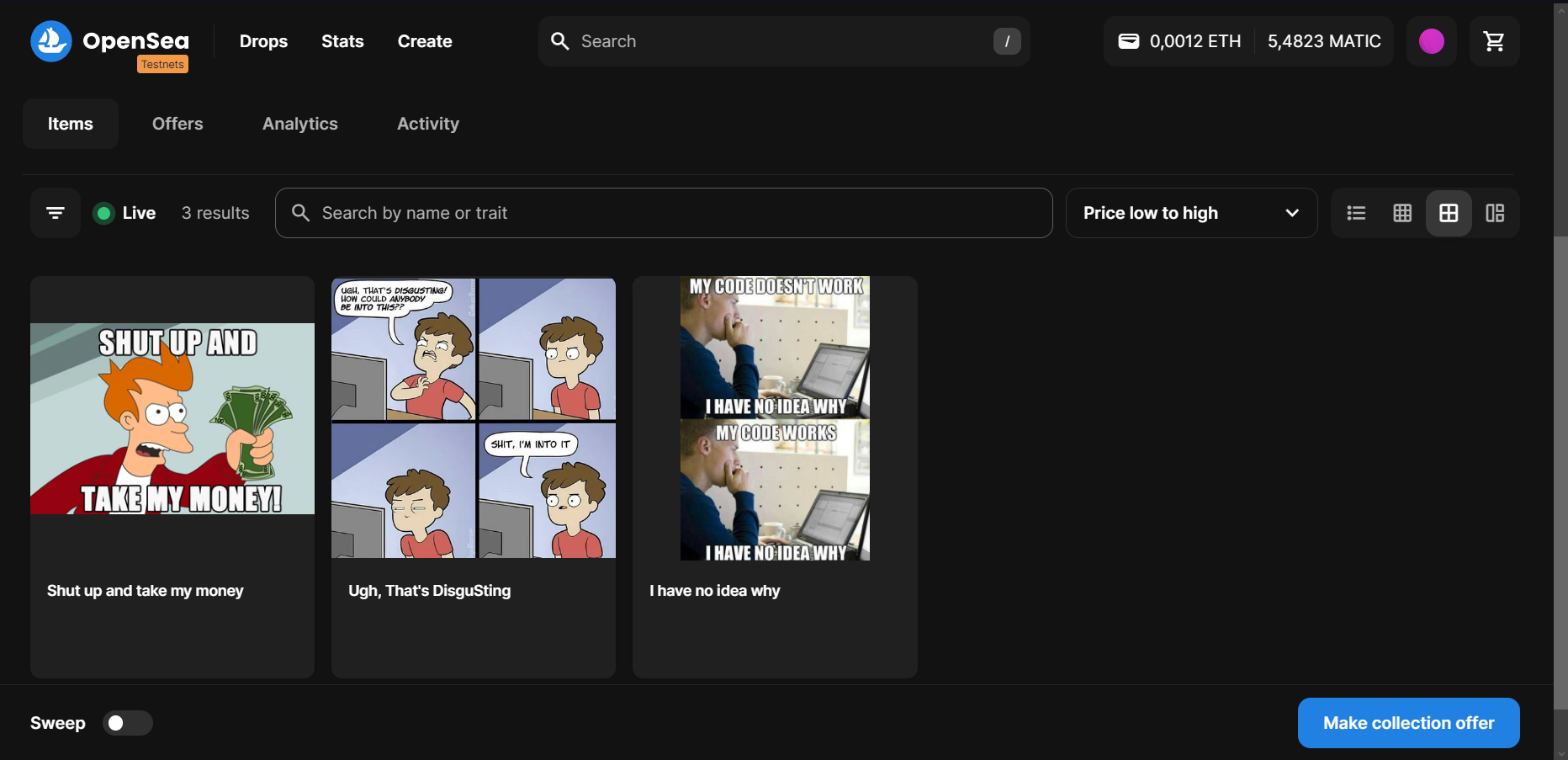